Permalink
Please
sign in to comment.
Browse files
Add method for determining binary file extension. (#349)
This PR implements the strategy described in the discussion of the above issue to get an extension for a file described by a URL and a MIME type. It creates a GetExtensionMime object in the matchbox. This PR also removes most of the filtering by URL from the image, audio, video, presentation, spreadsheet, and word processor document extraction methods, since these were returning false positives. (CSV and TSV files are a special case, since Tika detects them as "text/plain" based on content.) Finally, I have inserted toLowerCase into the getUrl.endsWith() filter tests, which could possibly bring in some more CSV and TSV files * Adds method for getting a file extension from a MIME type. * Add getExtensions method to DetectMimeTypeTika. * Matchbox object to get extension of URL * Use GetExtensionMime for extraction methods; minor fixes. * Remove tika-parsers classifier * Remove most filtering by file extension from binary extraction methods; add CSV/TSV special cases. * Fix GetExtensionMime case where URL has no extension but a MIME type is detected * Insert `toLowerCase` into `getUrl.endsWith()` calls in io.archivesunleashed.packages; apply to `FilenameUtils.getExtension` in `GetExtensionMime`. * Remove filtering on URL for audio, video, and images. * Remove filtering on URL for images; add DF fields to image extraction * Remove saveImageToDisk and its test * Remove robots.txt check and extraneous imports * Close files so we don't get too many files open again. * Add GetExtensionMimeTest * Resolve #343
- Loading branch information...
Showing
with
219 additions
and 258 deletions.
- +1 −36 src/main/scala/io/archivesunleashed/df/package.scala
- +25 −0 src/main/scala/io/archivesunleashed/matchbox/DetectMimeTypeTika.scala
- +1 −3 src/main/scala/io/archivesunleashed/matchbox/ExtractImageDetails.scala
- +58 −0 src/main/scala/io/archivesunleashed/matchbox/GetExtensionMime.scala
- +47 −105 src/main/scala/io/archivesunleashed/package.scala
- BIN src/test/resources/warc/example.media.warc.gz
- +0 −112 src/test/scala/io/archivesunleashed/df/SaveImageBytesTest.scala
- +1 −2 src/test/scala/io/archivesunleashed/df/SaveMediaBytesTest.scala
- +86 −0 src/test/scala/io/archivesunleashed/matchbox/GetExtensionMimeTest.scala
@@ -0,0 +1,58 @@ | |||
/* | |||
* Archives Unleashed Toolkit (AUT): | |||
* An open-source toolkit for analyzing web archives. | |||
* | |||
* Licensed under the Apache License, Version 2.0 (the "License"); | |||
* you may not use this file except in compliance with the License. | |||
* You may obtain a copy of the License at | |||
* | |||
* http://www.apache.org/licenses/LICENSE-2.0 | |||
* | |||
* Unless required by applicable law or agreed to in writing, software | |||
* distributed under the License is distributed on an "AS IS" BASIS, | |||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | |||
* See the License for the specific language governing permissions and | |||
* limitations under the License. | |||
*/ | |||
package io.archivesunleashed.matchbox | |||
|
|||
import org.apache.commons.io.FilenameUtils | |||
|
|||
/** Get file extension using MIME type, then URL extension. */ | |||
// scalastyle:off object.name | |||
object GetExtensionMime { | |||
// scalastyle:on object.name | |||
|
|||
/** Returns the extension of a file specified by URL | |||
* | |||
* @param url string | |||
* @param mimeType string | |||
* @return string | |||
*/ | |||
def apply(url: String, mimeType: String): String = { | |||
val tikaExtensions = DetectMimeTypeTika.getExtensions(mimeType) | |||
var ext = "unknown" | |||
// Determine extension from MIME type | |||
if (tikaExtensions.size == 1) { | |||
ext = tikaExtensions(0).substring(1) | |||
} else { | |||
// Get extension from URL | |||
val urlExt = FilenameUtils.getExtension(url).toLowerCase | |||
if (urlExt != null) { | |||
// Reconcile MIME-based and URL extension, preferring MIME-based | |||
if (tikaExtensions.size > 1) { | |||
if (tikaExtensions.contains("." + urlExt)) { | |||
ext = urlExt | |||
} else { | |||
ext = tikaExtensions(0).substring(1) | |||
} | |||
} else { // tikaExtensions.size == 0 | |||
if (!urlExt.isEmpty) { | |||
ext = urlExt | |||
} // urlExt = "" => ext = "unknown" | |||
} | |||
} | |||
} | |||
ext | |||
} | |||
} |
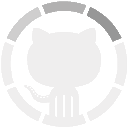
Oops, something went wrong.
0 comments on commit
448601e